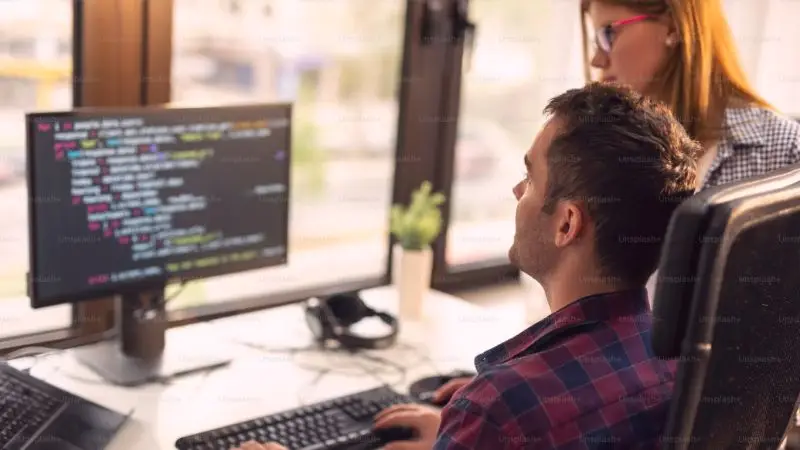
Many programmers aim to write clean, scalable and maintainable code in ReactJS. This skill separates good programmers from great programmers, as it is easy to fall into bad habits that can make code difficult to read and maintain.
There are a few essential practices that can help ensure that your code remains readable and usable for both yourself and other developers. Some consider these practices optional, but they can make a difference when used correctly.
Maintain good folder structure
React does not recommend a specific folder structure, but it offers some suggestions on its website. The best folder structure for your application will depend on its size and your team’s preferences.
When structuring your project, there are two main principles — avoid excessive nesting and don’t over-engineer it. Regardless of your chosen structure, ensure that your folder structure is consistent, maintainable and readable. Having a clean folder structure can make a big difference in the long run.
Keep proper code structure
There is no one-size-fits-all approach to structuring a React project, but some general principles can help you code better.
These principles include maintaining a structured import order, using consistent quotation marks, dividing the app into small components, avoiding repetitive codes (also known as the Don’t Repeat Yourself (DRY) principle) and using object destructuring for props.
Remember to follow common naming conventions, such as capitalizing your components. It can heavily impact the readability of your application.
Type check with PropType, TypeScript
PropType is a form of type-checking that React programmers can use in plain JavaScript. It can help prevent unexpected and hard-to-debug behavior by passing the correct data into components.
TypeScript is another way to achieve this, as it can help reduce the number of unexpected bugs you and other developers encounter. It also provides better code completion in your IDE, making writing code quickly and accurately easier. However, if your project is small, using TypeScript can be a waste of time instead.
Use linting tools
A linter is a tool that analyzes your JavaScript code and identifies potential errors. Linters can help you write more consistent, readable and error-free code. They can also be helpful when working with a team of developers, as they can ensure that everyone is following the same coding style. ESLint is a popular linter that programmers can use with React.
Keep display and business logic separate
It is best to keep your stateful data-loading logic separate from your rendering logic. It will make your components simpler and easier to debug or refactor.
For example, if your application makes an API call, you can manage the API call in a parent component and pass the data to the element to be rendered via props. This will make your code easier to understand and more readable.
Make test cases
Testing is often overlooked in React projects, but it is a valuable practice that can save time and improve the quality of your code. By writing tests, you can catch errors early in development and prevent them from causing problems later.
There are three main types of testing for React projects — unit testing, component testing and end-to-end (E2E) testing. Unit tests test individual units of code, component tests test entire components and E2E tests test the whole application from start to finish.
It is recommended to start with unit testing and then move on to component and E2E testing. By having a well-tested codebase, you can be confident that your application will work as expected and that you can add new features without introducing new bugs.